Leonids is a particle system library that works with the standard Android UI. It is Free Software and is available on GitHub.
You can download Leonids Demo from Google Play to check out what can be done with it.
The library is extremely lightweight, it is just a jar file of 18Kb you can add to your project. You can download LeonidsLib.jar from the project page on GitHub.
Why this library?
Particle systems are often used in games for a wide range of purposes: Explosions, fire, smoke, etc. This effects can also be used on normal apps to add an element of “juiciness” or Playful Design.
Precisely because its main use is games, all engines have support for particle systems, but there is no such thing for standard Android UI.
This means that if you are building an Android app and you want a particle system, you have to include a graphics engine and use OpenGL -which is quite an overkill- or you have to implement it yourself.
Leonids is made to fill this gap, bringing particle sytems to developers that use the standard Android UI.
Code examples and features
You can get the code for all the examples on GitHub, but let’s get to the basics.
Simple one shot
Creating and firing a one-shot particle system is very easy, just 3 lines of code.
new ParticleSystem(this, numParticles, drawableResId, timeToLive)
.setSpeedRange(0.2f, 0.5f)
.oneShot(anchorView, numParticles);
When you create the particle system, you tell how many particles will it use as a maximum, the resourceId of the drawable you want to use for the particles and for how long the particles will live.
Then you configure the particle system. In this case we specify that the particles will have a speed between 0.2 and 0.5 pixels per milisecond (support for dips will be included in the future). Since we did not provide an angle range, it will be considered as “any angle”.
Finally, we call oneShot, passing the view from which the particles will be launched and saying how many particles we want to be shot.
This produces a fireworks-like effect as you can see here:
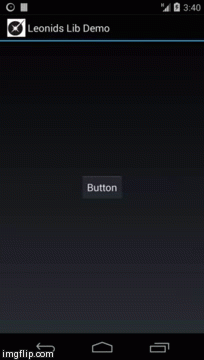
One Shot – Dust simulation
As an example of something used in production, this dust simulation is almost identical to the one implemented on the game Rabbit and Eggs, but made with Leonids instead of AndEngine.
new ParticleSystem(this, 4, R.drawable.dust, 3000)
.setSpeedByComponentsRange(-0.07f, 0.07f, -0.18f, -0.24f)
.setAcceleration(0.00003f, 30)
.setInitialRotationRange(0, 360)
.addModifier(new AlphaModifier(255, 0, 1000, 3000))
.addModifier(new ScaleModifier(0.5f, 2f, 0, 1000))
.oneShot(findViewById(R.id.emiter_bottom), 4);
It sets an initial rotation range and then 2 modifiers for Alpha and Scale. Using modifiers is the advanced way of managing a particle system. It allows more flexibility about the starting and end times.
The parameters for the modifiers are: initialValue, endValue, startTime and endTime. In this case, we do a scale from 0.5 to 2 in the first 1.5 seconds and a fade out from second 1 to 3.
We also set an external acceleration simulating wind.
And it looks almost exactly like the one in the game, but on a standard Android UI:
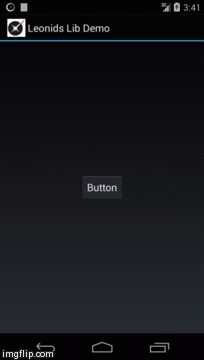
Using emitters – Confeti
Another example we used on Rabbit and Eggs was confeti. This is done using two emitters one on each side of the top of the screen
new ParticleSystem(this, 80, R.drawable.confeti2, 10000)
.setSpeedModuleAndAngleRange(0f, 0.3f, 180, 180)
.setRotationSpeed(144)
.setAcceleration(0.00005f, 90)
.emit(findViewById(R.id.emiter_top_right), 8);
new ParticleSystem(this, 80, R.drawable.confeti3, 10000)
.setSpeedModuleAndAngleRange(0f, 0.3f, 0, 0)
.setRotationSpeed(144)
.setAcceleration(0.00005f, 90)
.emit(findViewById(R.id.emiter_top_left), 8);
Which looks like this:
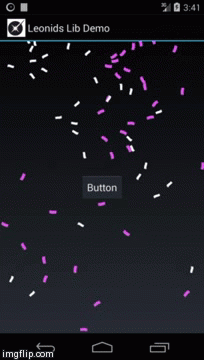
Other details
Leonids requires minSDK 11 because it uses ValueAnimators. It should be very easy, however to use the compatibility library and make it work on Gingerbread.
The library is Free Software, you can use it, extended with no requirement to open source your changes. You can also make paid apps using it.